Download Files
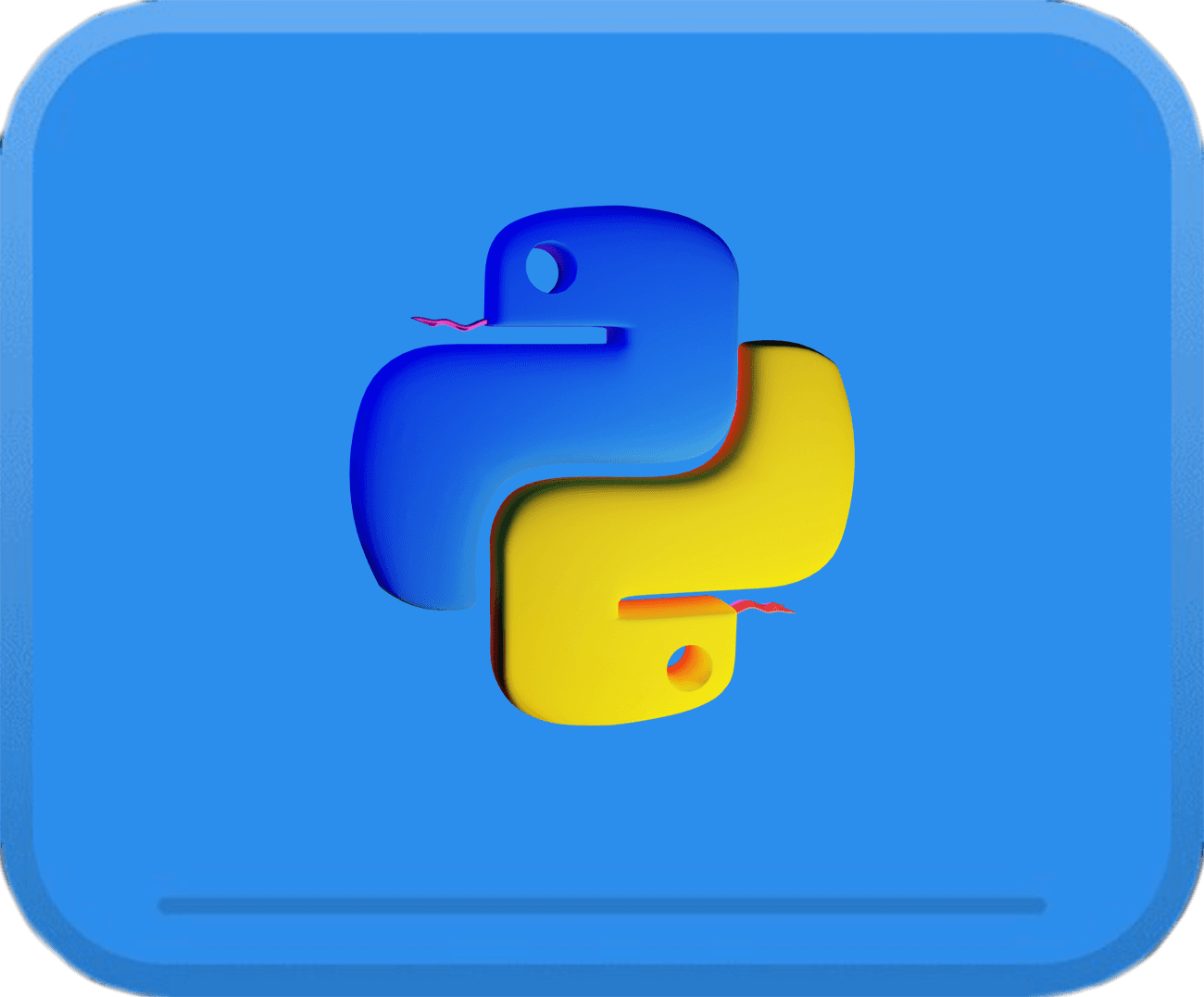

Resources
Summary
Intro
Let's learn how to work with ViewTemplates and regular Views in Revit API. When you compare the two, you will realize that they both use the same View Class. The only difference is that there is a property called IsTemplate, which defines if it's a regular view or a ViewTemplate. So nearly everything you will learn in this lesson can be applied to both Views and ViewTemplates.
In this lesson you will learn How to:
Get All ViewTemplates
Sort Views by ViewTemplates
Assign / Remove ViewTemplates
Modify ViewTemplate Parameters
Modify ViewTemplate Properties
Modify Category Visibility or Overrides
Modify Workset Visibility
Hide/Unhide/Isolate Elements in View
💡I also wanted to show how to Transfer ViewTemplates between projects, but the lesson became too long… So you will learn about it in the next lesson.
Get All ViewTemplates
Let's begin with the basics and get all ViewTemplates.
As I've mentioned, Views and ViewTemplates share the same Class, so we can get them with FEC using OfClass or OfCategory. This will give you a list of both Views and ViewTemplates.
Now we can filter only ViewTemplates in a list comprehension using the IsTemplate property.
Here is the code to filter only Views or ViewTemplates:
Sort Views by ViewTemplates
Now, let's look at how to sort our views by ViewTemplates.
We will copy the first step from the previous example to get all views and view templates.
Then, I want to create a dictionary with ViewTemplate.Name
as a key and a list_of_views
that use that view templates as a value.
e.g. {VT.NAME : [view_1, view_2…]}
We have to do the following Steps:
Create defaultdict(list) - This will avoid the error when key is not in the dict yet.
Iterate through Views and get ViewTemplateId
Ensure that ViewTemplateId is not equal to ElementId(-1) (meaning None in Revit)
Get ViewTemplate Name or use 'No Viewtemplate' to add views to a dictionary
Here is the Code:
Assign/Remove ViewTemplate
Alright, what if we want to assign or remove ViewTemplates?
This is very simple, because as you've already noticed there is a property called ViewTemplateId. This property is both readable and writable. So, we can just use it to assign another ViewTempalte by providing its Id like:
view.ViewTemplateId = vt.Id
And if you want to remove the ViewTemplate, then you would need to provide ElementId(-1), because it represents None.
Modify View Templates
Now, let's have a look how to modify our ViewTemplates and Views.
First of all, let's prepare the code where we will get the ActiveView and ensure that it has an active ViewTemplate. Otherwise, we will create an alert message with pyrevit.forms.alert
😉 Also, keep in mind that we will be making changes to our project, so don't forget to use the Transaction and write all the next steps between Start and Commit statements.
Modify Parameters / Properties
Now, let's begin with changing ViewTemplate Parameters and Properties.
You will notice that there are some parameters that can also be overridden by using properties. Personally, I prefer to use properties when possible, and I use parameters only for shared View Parameters. However, you can always check if parameters are ReadOnly or not.
But pay attention if your views have a ViewTemplate assigned or not, because it will make your shared parameters ReadOnly, as it's controlled by View Template.
Let's change a parameter in a ViewTemplate. I will use the shared parameter: 'Browser Organisation Ebene 2'. You can add any shared or project parameter to your views and test it.
Also, I will change Properties DetailLevel and Scale.
When you are not sure, you can check these properties in the Revit API Docs to understand if you can override them and what kind of Type do you need to provide.
In case of DetailLevel, you can see that you can SET this value, and it returns ViewDetailLevel,
therefore you know that it's also the type you have to use to set the value.
💡Do not mistake View with ViewTemplate in your code.
It's very common mistake to try to change View's properties or parameter values when they have a ViewTemplate assigned.
It can be because either we haven't checked the ViewTemplate and all View's parameters became ReadOnly. Or just because we used the wrong variable in the code (active_view / active_vt)
So keep this in mind, especially when you see this error message.
Set Category Hidden
Now, let's look at how to modify Graphic/Visibility Settings of our Views or ViewTemplates.
There is a method SetCategoryHidden, and it needs an ElementId of a Category and a True/False boolean to hide or not.
We can use GetCategory to get Walls, and then use it to change it's visibility setting like this:
Set Category Overrides
In case you would want to change the color overrides of your categories, you would need to use SetCategoryOverrides, and it takes Category.Id, and OverrideGraphicSettings.
You already know how to create these settings from the previous lesson.
Here is the code sample:
Set Workset Visibility
Also, we can control visibility of the worksets. It's very simple to use SetWorksetVisibility, however it might be a bit tricky to get all worksets for the first time.
To get all worksets, you need to use FilteredWorksetCollector. It also takes the doc argument, but then you would use OfKind Method and provide WorksetKind Enumeration.
Here is an example.
Hide Elements
Lastly, let's have a look how to hide or unhide elements in our views. Overall, it's fairly straight-forward.
For this example, I will use FEC class to get all Furniture instances in my project. Then we need to ensure that all these elements CanBeHidden and convert it to List[ElementId].
Lastly, we just use HideElements method on the view and provide that list.
Isolate Elements
Sometimes we might want to isolate elements with Revit API, but there isn't such method…
So instead of that, we need to hide all elements except for those that should be isolated. And we can use ExclusionFilter for that.
So it will work like this:
Get Element_Ids to Isolate
Create ExclusionFilter
Get All Elements except for Isolate Elements with FEC
Ensure Elements CanBeHidden
Hide all other elements
Here is the Code:
Unhide + Isolate Elements
Lastly, I want to show you how to unhide your elements.
Not only it can be a much quicker way to unhide everything in a view, it can also be used when you want to isolate your elements.
💡 If something was already hidden before you started to isolate it, it will not be shown in the end…
So, therefore, it might be a good idea to unhide everything first, and then isolate your elements, so you can actually see everything that you wanted.
To do that we just need to get ElementIds of all elements that CanBeHidden and use UnhideElements like this:
How to control Include Parameter in ViewTemplate?
I've forgotten to mention during the video, but if you want to control what parameters are included or not in the ViewTemplate here is what you would need to use:
GetTemplateParameterIds - Returns a list of parameter ids that may be controlled when this view is assigned as a template.
SetNonControlledTemplateParameterIds - It sets the parameters that will not be included when this view is used as a template.
You can read more about this in this forum discussion. It will give you a better idea on how to handle this Include option.
You can also check this code snippets by Andreas Draxl.
Huge thanks for sharing it with us!
Summary
Hopefully, these examples will inspire you to create cool new tools for your office to handle Views and View Templates better.
There are a lot more things we could do with our Views, so let me know in the community if you have any more questions.👀👇
HomeWork
Practice working with Views in Revit API.
I hope that you had some inspiration during the lesson of what you could do with your Views and ViewTemplates.
If not, here is a simple exercise to try it out yourself:
Get All View Templates
Filter ViewTemplates that use 1:50 Scale
Hide all Categories except for Floors and Walls (How to get all cats?)
Get all related Views
Create a Report (Print views with this ViewTemplate)
P.S. If you want to get all categories, you can use this snippet:
⌨️ Happy Coding!