Resources
Summary
Key Schedule Parameters
You know that we can create Key-Schedules that creates a parameter for our elements. And if you inspected it with Revit Lookup you might have noticed that they are a little more tricky, because their StorageType is ElementId.
That means that we need to get all possible values and then provide ElementId of a specific value that we want to assign.
I think Key-Schedules are most common to use on Rooms, but people won't stop surprise me how they use Revit. So feel free to share how you use Key-Schedule in your work!
Create Key-Schedule
Let's start by creating a Key-Schedule for rooms.
I will leave my Key Name as Room Style for this lesson and we will fill some random values so we can test them with Revit API.
Explore Key Parameter
Once we prepared our Revit we can dive deep and look inside our elements.
Our rooms now have a new Key-Parameter called Room Style, so let's look inside of it with Revit Lookup.
We are going to Snoop Selected Room, and look for Room Style in Parameters.
You will notice that this parameter has StorageType.ElementId, because it's refferenced to item sin Key ViewSchedule.
Unfortunately, there is no property that would identify this parameter as a Key-Parameter. We either have to know this before we begin, or go through our schedule and find matching parameter name…
We can use ViewSchedule's Property
.KeyScheduleParameterName to identify that it's a Key Schedule.
Explore Key-Schedule
We know that everything leads us back to Key-Schedule, so it's worth opening it and exploring with Revit Lookup as well.
When you have your Key-Schedule as an ActiveView, we can use Snoop Active View option in Revit Lookup.
That's a quick and easy way to look inside of our views to better understand Revit API
Once you are inside the view you can scroll through and see if anything indicates that it's a Key schedule and what is the Key Parameter Name.
And you will find property: KeyScheduleParameterName.
In our case it says Room Style, but for non-key-schedules it will be None here.
That's how we know if it's a key-schedule.
What do we need to change Key-Parameter value?
If we want to change values of our Key-Parameters we need to provide ElementId of matching values. But the question now, how do we get these values?
There is a quick and easy way to do that by using FilteredElementCollector(FEC).
It's a class that allows us to get elements from our projects based on filtering criteria we create. It's not complicated, but it might look intimidating first time you see it.
💡 You will learn how to use it in the Module 06, but for now I will keep it simple.
Get Key-Schedule Values with FilteredElementCollector.
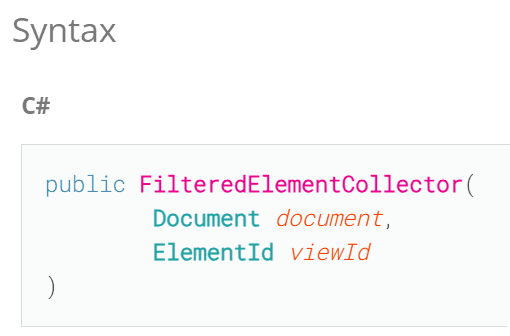
To do this, we will need to use second constructor of FEC Class.
It takes 2 arguments:
document - from where we are getting Elements
view_id - Id of a View where we get visible elements
This allows us to provide ElementId of a view, from where we are trying to get elements. And in the case - ViewSchedule will only return Key-Values as found elements.
👇 Here is the snippet to get them if you have Key-Schedule as View Id.
💡You can simply test it using RevitPythonShell. Here are results.
Prepare a button
I think I found all the pieces of the puzzle, now it's about combining it together in a code that we could use.
It will be broken down into 2 Steps:
1️⃣ Get all Key-Parameter Values
2️⃣ Change Key-Parameter Value
📁 Create a button for this lesson and let's start Coding!
Get Key-Parameter Values
Next we are going to get all matching Key-Parameter values.
We can break it down into 3 steps:
1️⃣ Get All Schedules using FilteredElementCollector
2️⃣ Find ViewSchedule with matching KeySheduleParameterName
3️⃣ Display Name and Id values of Key-Values
👀 Here are the results if you run this Code Snippet to get Key-Values

🎦 It would be better if you follow the video when I write this code to better understand
Change Key-Parameter Value
✅ Now we have our Key-Parameter Values.
We just need to select values that we want and apply it using Set method of Parameters by providing correct ElementId.
You already know this from previous lessons, but here is an example as well.
HomeWork
To be honest, Key-Schedules are not as common as regular parameters. So this time you can decide if you need to practice it yourself.
💡 I would recommend you to follow the steps in the lesson if you want to really understand what is going on.
📅 But you can also leave it until the day you need to work with Key-Schedules, and then you can come back and refresh your memory by following this Lesson again!
⌨️ Happy Coding!