Last Chance To Claim LIFETIME ACCESS. [~40 Seats left]
Resources
Jul 25, 2024
pyRevit WPF Form for Asking user for Multiple Paths
If you ever wanted to ask your users for multiple paths, you can use this custom WPF form.
EF-WPF Form
Here is the overview of the form that I will give you the code for.
It's simple, but yet very elegant to collect multiple paths. But also you can extend the functionality if you know how to work with WPF.
Happy Coding!
Folderstructure
To create WPF forms you need to create .xaml file and then use it inside of your python script.

Let's break them down one by one.
.XAML Script
Xaml - is the file format for WPF forms, which is used for designing front-end of the forms.
In this case you have to create a file FormFolderPaths.xaml
in the same folder as yourscript.py
, because I will be referencing the name of the file inside the python file.
Here is the code:
Script.py
And here is the python file with my template
Results

Join Newsletter
📩 You will be added to Revit API Newsletter
Join Us!
which is already read by 7200+ people!





Ready to become Revit Hero for your office? Learn Revit API!
Join this comprehensive course that will guide you step by step on how to create your dream tools for Revit that save time.
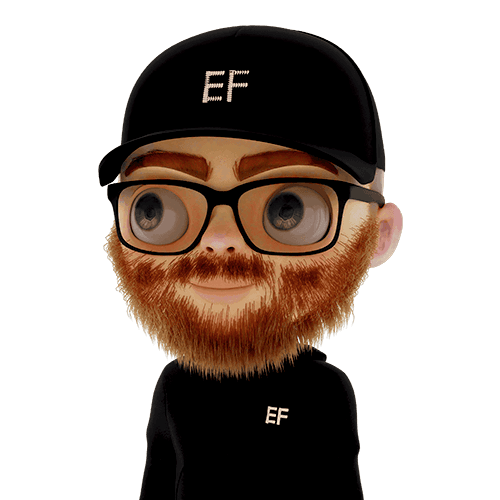