Dec 25, 2023
Get Elements with ElementParameterFilter
Here you will learn about getting Elements,Type or Family by providing a Type or Family Name.
Filter Elements by parameter Value
If you are a Revit API enthusiast, then at certain point you wanted to get your elements or types with a parameter value. Foe example get all instance of a specific Type or Family Name. It sounds like a very logical thing that should be within Revit API, but it's not.
So let me help you with that! Below I will provide a brief explanation on how to make a function to get elements by Type or Family name using ElementParameterFilter Class.
And also you will learn how to modify it for any other parameter!
Get Element of a certain Type Name
We will use ElementParameterFilter to create a function to get elements by Type Names.
It needs:
Parameter Id to create ParameterValueProvider
Evaluator (FilterStringEquals) in this case
Also notice that there were changes in Revit API for this class so I will account for that too
Then we can provide all of this to create ElementParameterFiler
And we can use this filter for FilteredElementCollector with WherePasses
Get Elements with Family Name
We can also modify very little a get elements by Family name instead of type.
To do that we need to
- Change Parameter Id to BuiltInParameter.ALL_MODEL_FAMILY_NAME
- Rename function and variables
The rest stays the same, here is complete Snippet.
Get Types by Type or Family Name
You might also want to get types instead of instances and you can use exact duplicates of these functions but replace
Here is an example:
ElementParameterFilter
These are examples on how to use ElementParameterFilter.
You can adjust very little and it will work with other parameters, and evaluators.
There will be a post about it in more detail in the future 😉
Join Newsletter
📩 You will be added to Revit API Newsletter
Join Us!
which is already read by 7500+ people!





Ready to become Revit Hero for your office? Learn Revit API!
Join this comprehensive course that will guide you step by step on how to create your dream tools for Revit that save time.
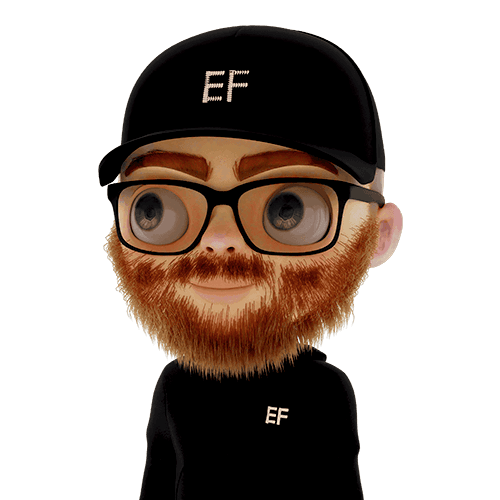