Jun 10, 2024
Create Interactive Revit Reports with pyRevit Linkify
Learn how to use pyRevit's Linkify to create linkable buttons to select elements, open views or even find elements in the active view
pyRevit Linkify
pyRevit Linkify is a great feature that can create a linkable buttons to your elements and it can do 3 things:
- Select Elements
- Open Views
- Find Elements in the ActiveView (Zoom on it)
And it's super easy to use! Let's have a look at the documentation first to understand how to use it.
pyRevit Docs
There are 2 places you can check:
1. pyRevit Dev Docs - Effective Outputs

2. pyRevit ReadTheDocs - Linkify
Import Linkify

Before we dive into the examples, I want you to understand that you need to import script from pyRevit and then get the output so we can use linkify function. Here is the example:
Now let's have a look at a few examples.
Example 1 - Linkify Single Elements
Let's begin by looking at the examples.
Here is an example to create Linkify for a single element. We will grab all walls in a view and then create linkify button for each wall. Also notice that I've provided wall.Name
as the second argument, and that will be the title of our linkify button.
Here is the code:

Example 2 - Linkify Multiple Elements
We can also provide a list of Element Ids if we want to create a linkable button for many elements at once.
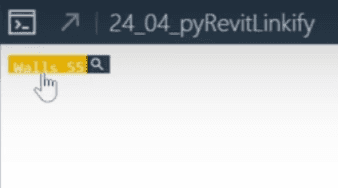
Example 3 - Linkify Limitation
But keep in mind that Linkify has a limit on how many elements can be included in a single button. Usually you will notice it somewhere around 100-150 elements.
Let's put hundreds of walls into a single linkify button to see what kind of an issue we might encounter.
This will still create the button without any issues, however, when you try to use it you will see the following error:

Example 4 - Linkify Views
You will also notice that you can linkify views. And by clicking on suck a linkify button it will open that specific view.
It's great if you want to quickly create a list of views and easily jump between them. This is especially useful if you work on a large project and you have hundreds of views, and sometimes it gets tedious to open the right ones for the day.
So here is a simple sample:
Example 5 - Analyze Warnings
Alright, now let's put this to use.
You've seen various example of using Linkify, but probably it wasn't that impressive. Now I want to show you a simple tool that can be extremely useful when you work with Revit Warnings.
This example will:
1. Get all warnings in the project,
2. Find elements related to these warnings
3. Find out who was the last person to modify them.
4. Print out the table that will have
- Warning Description
- Username of LastChangedBy
- Linkable Button to select and find these elements
Overall it's not that complicated, and it gives you great results like this:

Join Newsletter
📩 You will be added to Revit API Newsletter
Join Us!
which is already read by 7500+ people!





Ready to become Revit Hero for your office? Learn Revit API!
Join this comprehensive course that will guide you step by step on how to create your dream tools for Revit that save time.
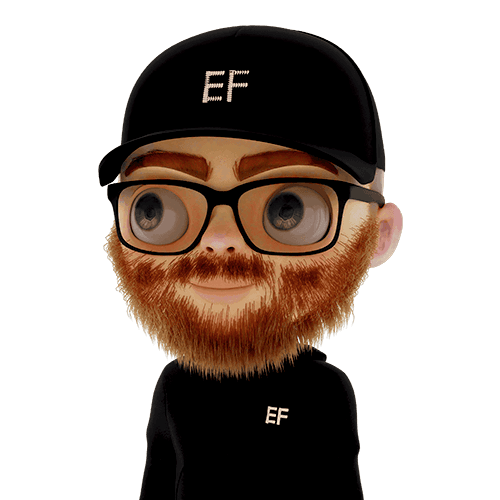