What is Revit API Events or pyRevit hooks?
Event - Is an action that can be monitored by the software. This means that we can subscribe to an event, and whenever it happens we will execute a piece of our code.
pyRevit hook is a simplified way of subscribing to Events. It allows you to simply create hooks folder, and name your python file and pyRevit knows what to do next.
Why do we need Events?
When we work with Revit API we have an ability to subscribe to Events, and execute code when they are triggered. This allows you to wait for certain actions and then execute your code when it happens. It can open a whole new world of automation in Revit.
For example, we know that Import CAD is a cursed button, and many BIM managers try very hard to make everyone Link CAD files instead of importing because they bring a lot of useless patterns, and don't have an original path associated with them… So we can subscribe to a command event of Import CAD button, and whenever it's clicked we can execute our assigned code (show a warning message in this case).
This examples is exactly what I will show you how to create by the end of this lesson. But first let's explore Revit API events a bit more.
Revit API Event Types
There are a lot of events available in Revit API, and I will try to categorize and list them here starting with Database events.
1️⃣ DataBase Events (Application )
Database events are looking for changes related to the project and application state. It will monitor when projects are being saved, opened printed, exported and similar interactions. These events can be found under Application Class and here is a list of them:
DocumentChanged - notify when a transaction is committed, undone or redone
DocumentClosing - notify when Revit is about to close a document
DocumentClosed - notify just after Revit has closed a document
DocumentCreating - notify when Revit is about to create a new document
DocumentCreated - notify when Revit has finished creating a new document
DocumentOpening - notify when Revit is about to open a document
DocumentOpened - notify after Revit has opened a document
DocumentPrinting - notify when Revit is about to print a view or ViewSet of the document
DocumentPrinted - notify just after Revit has printed a view or ViewSet of the document
DocumentReloadedLatest - notify after Revit has finished reloading a document with central model
DocumentReloadingLatest - notify when revit is about to reload latest changes from central model
DocumentSaving - notify when Revit is about to save the document
DocumentSaved - notify just after Revit has saved the document
DocumentSavingAs - notify when Revit is about to save the document with a new name
DocumentSavedAs - notify when Revit has just saved the document with a new name
DocumentSynchronizingWithCentral - notify when Revit is about to synchronize a document with the central file
DocumentSynchronizedWithCentral - notify just after Revit has synchronized a document with the central file
DocumentWorksharingEnabled - notify when a document has become workshared
ElementTypeDuplicated - notify when Revit has finished duplicating an element type.
ElementTypeDuplicating - notify when Revit is just about to duplicate an element type.
FailuresProcessing - notify when Revit is processing failures at the end of a transaction
FamilyLoadedIntoDocument - notify after Revit loaded a family into a document.
FamilyLoadingIntoDocument - notify when Revit is just about to load a family into a document.
FileExporting - notify when Revit is about to export to a file format supported by the API
FileExported - notify after Revit has exported to a file format supported by the API
FileImporting - notify when Revit is about to import a file format supported by the API
FileImported - notify after Revit has imported a file format supported by the API
LinkedResourceOpened notify immediately after Revit has finished opening a linked resource.
LinkedResourceOpening notify when Revit is just about to open a linked resource.
ProgressChanged - notify when an operation in Revit has progress bar data
ViewExported notify immediately after Revit has finished exporting a view of the document.
ViewExporting notify when Revit is just about to export a view of the document.
ViewPrinted - notify just after Revit has printed a view of the document
ViewPrinting - notify when Revit is about to print a view of the document
ViewsExportedByContext notify immediately after Revit has finished exporting one or more views of the document via an export context by CustomExporter.
ViewsExportingByContext notify when Revit is just about to export one or more views of the document via an export context by CustomExporter.
WorksharedOperationProgressChanged notify when progress has changed during Collaboration for Revit's workshared operations: open model and synchronize with central.
Then we have related to Revit UI. These are about interaction with the application or certain actions being taken. All these events are located in UIApplication Class, and here is the list:
ApplicationClosing -be notified when the Revit application is just about to be closed.
DialogBoxShowing - be notified when Revit is just about to show a dialog box or a message box.
DisplayingOptionsDialog - be notified when Revit options dialog is displaying.
DockableFrameFocusChanged - be notified when a Revit GenericDockableFrame has gained focus or lost focus in the Revit user interface.
DockableFrameVisibilityChanged - be notified when a Revit GenericDockableFrame has been shown or hidden in the Revit user interface.
FabricationPartBrowserChanged - be notified when MEP Fabrication part browser is updated.
FormulaEditing - be notified when the edit formula button has been clicked.
Idling - be notified when Revit is not in an active tool or transaction.
SelectionChanged - be notified after the selection was changed.
TransferredProjectStandards - be notified after the scope of a Transfer Project Standards operation has been finalized.
TransferringProjectStandards - be notified before the scope of an impending Transfer Project Standards operation has been finalized in the Transfer Project Standards dialog.
ViewActivated - be notified immediately after Revit has finished activating a view of a document.
ViewActivating - be notified when Revit is just about to activate a view of a document.
There are also Events under Autodesk.Revit.UI - PreviewControl for monitoring user's interaction like DragEnter, DragLeave…
3️⃣ CommandBinding Event
We can create Command Binding Events. This will allow us to monitor commands within Revit and subscribe to them. You can see a list of all commands in PostableCommand Enumeration, it has hundreds if not thosands of commands, so I won't list them here…
On top of that all these commands can be subscribed as:
BeforeExecuted- This read-only event occurs before the associated command executes. An application can react to this event but cannot make changes to documents, or affect the invocation of the command.
CanExecute - Occurs when the associated command initiates a check to determine whether the command can be executed on the command target.
Executed - This event occurs when the associated command executes and is where any overriding implementation should be performed.
💡pyRevit simplifies this step for us, but I will also show you how to manually subscribe with code, and these stages of an Event will matter.
4️⃣ IUpdater (Dynamic Model Update)
Lastly, I want to mention that there is also an IUpdater Interface. It's The interface used to create an updater capable of reacting to changes in the Revit model. It also has its hook equivalent in pyRevit doc-updater.py
Technically it's not an event, but it acts like one. It allows us to monitor changes in the project, and piggy back on the same Transaction to add our additional changes to it.
Just to give you an example how it can be used:
We can monitor changes for Windows, and whenever they change, we might want to update X,Y,Z coordinates in one of the parameters, so we can actually tag their location and it will always update as you move them.
There are endless possibilities for new functionality by using IUpdater. It's incredible useful, but I won't cover it in this lesson. It deserves a lesson of its own. Comment on the YouTube video if you want to see how to use it 😉
So how do we work with Events?
We have 2 options to subscribe to Events in Revit API.
🅰️ pyRevit Hooks - Very powerful and beginner friendly approach
🅱️ Manually Subscribe to Events - You can also write the whole code yourself to subscribe, but then it needs to be executed every time you start Revit or whenever you need it.
I will mainly focus on pyRevit hooks, but I will also show an example of doing it manually in the end.
🎯 Let's create an Event to monitor when users click on Import CAD button. And then we will give them a warning, saying that they should Link instead of importing and ask for a password, so only a select few users can import CAD files. You can apply the same concept to other buttons or other events, it's just an example I chose.
pyRevit Hooks
pyRevit provides a very simple approach to events. I will also link additional resources here that will help you understand them and see a few examples:
In a nutshell - you need to create a hooks folder in .extension folder and place your scripts for certain events. And keep in mind that naming of your scripts in that folder is very important. The name of the files will tell pyRevit what kind of event should it be subscribed to. You can see a table of all hooks in Extension Hooks.

Creating pyRevit hooks is not that complicated. It's all about creating a python file with the correct name, and writing the script that you want to execute when event gets triggered.
💡 I know it still sounds confusing, but it's not! Let me show you how to actually use it.
How to create pyRevit Hooks? (Example)
There are plenty of different hooks to choose from, but I will start with command-before-exec[<cmd-id>]
. This kind of hook can subscribe to certain commands inside of Revit and trigger BEFORE it's executed.
You can see a full list of commands and how to get them in the end of this post, but I need to name it command-before-exec[ID_FILE_IMPORT].py,
so it gets triggered when user click on Import CAD button.
Let's make sure our users won't import CAD to Revit Projects and only allow in Family Documents.

First of all we need to create a hook python file in a hooks folder. Make sure you name your file correctly. In my case I want to create an Event for ID_FILE_IMPORT command, so I will have to name my file command-before-exec[ID_FILE_IMPORT].py
Here is folder structure example: (.tab and lib folders are just for reference so you know where to place hooks))
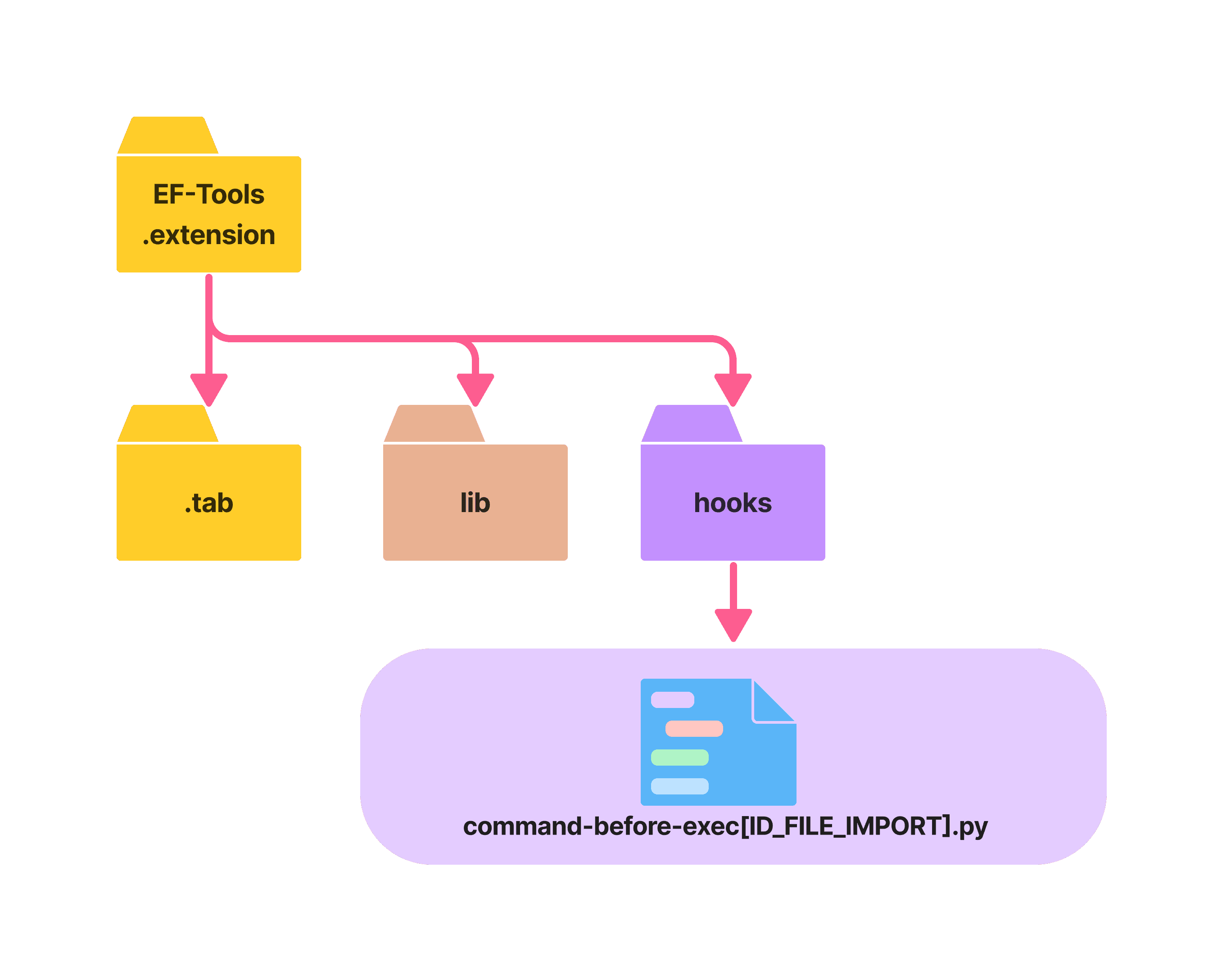
💡This file in hooks folder will tell pyRevit that the script inside should subscribe to ID_FILE_IMPORT command.
And here is a simple code we are going to put inside this python hook.
Whenever a user clicks on Import CAD, it will check if Document is project or family document, and if it's a project doc, then show a warning asking to use Link CAD. Additionally, it will also prompt a TextDialog asking for a password. If they enter the right one, they will be able to import CAD inside the project. Otherwise it won't allow importing CAD, and our BIM Managers can sleep tight at night.
from pyrevit import revit, EXEC_PARAMS
from Autodesk.Revit.UI import TaskDialog
sender = __eventsender__
args = __eventargs__
doc = revit.doc
if not doc.IsFamilyDocument:
TaskDialog.Show('Big Brother is Watching!',
'Import CAD is not Allowed! Use Link CAD Instead.')
from pyrevit.forms import ask_for_string
password = 'LearnRevitAPI.com'
user_input = ask_for_string(prompt='Only users with a password can Import CAD.',
title='Import CAD Blocked')
if user_input != password:
args.Cancel = True
else:
TaskDialog.Show('Family CAD Import',
'Import CAD is Allowed in families!')
👀 Here is the Result of how it works in a Project. You will notice that once I write the correct password it allows me to Import CAD. But if I would write it wrong, it would not stop the command.

How to get PostableCommand Id?
Let's go a step back and figure out how did I know that I need to use ID_FILE_IMPORT Command name for Command Binding?

I've noticed that people get very creative when looking for the right name of the command ID. You can find it in your journals or even in the .xml file of your shortcuts.
But we can also use LookupPostableCommandId Method which comes from Autodesk.Revit.UI.RevitCommandId.

This method takes a PostableCommand Enumeration, which has a list of all possible commands. There are hundreds if not thosands of them.

Here is an example of how to use it:
from Autodesk.Revit.UI import RevitCommandId, PostableCommand
all_post_commands = PostableCommand.GetValues(PostableCommand)
for pc in all_post_commands: #type: PostableCommand
try:
rvt_com_id = RevitCommandId.LookupPostableCommandId(pc) #type: RevitCommandId
print('{} {}'.format(str(pc).ljust(45), rvt_com_id.Name))
except:
print(pc)
This will give you a list of all Postable Commands.
RoofByFace ID_ROOF_PICK_FACES
Soffit ID_CREATE_SOFFIT_TB
LoadAsGroupIntoOpenProjects ID_LOAD_INTO_PROJECTS
LoadIntoProject ID_LOAD_INTO_PROJECTS_REBAR_SHAPE
RepeatComponent ID_REPEAT_COMPONENT
ConnectionSettings ID_STRUCTURAL_CONNECTION_SETTINGS
StructuralConnection ID_STRUCTURAL_CONNECTION_DIRECT_CREATION
ShowWarningsInViews ID_TOGGLE_STRUCTCONNECTIONS_SHOW_WARNINGS
ViewList ID_NEW_VIEWLIST
LinearDimensionTypes ID_SETTINGS_DIMENSIONS_LINEAR
AngularDimensionTypes ID_SETTINGS_DIMENSIONS_ANGULAR
SplitElement ID_SPLIT
SpotElevation ID_SPOT_ELEVATION
SpotCoordinate ID_SPOT_COORDINATE
AlignmentStation ID_ALIGNMENT_STATION_LABEL
AlignmentStationSet ID_ALIGNMENT_STATION_LABEL_SET
RoofByFootprint ID_ROOF_FOOTPRINT
RoofByExtrusion ID_ROOF_EXTRUSION
RadialDimensionTypes ID_SETTINGS_DIMENSIONS_RADIAL
ReloadLatest ID_WORKSETS_RELOAD_LATEST
Render ID_PHOTO_RENDERING_VIEW
ShowHistory ID_PARTITIONS_SHOW_HISTORY
SplitSurface ID_SPLIT_SURFACE
DrawOnFace 2706
DrawOnWorkPlane 2707
GrayInactiveWorksets ID_GRAY_INACTIVE_WORKSET_GRAPHICS
ImportGBXML ID_ABS_IMPORT_GBXML
ExportReportsRoomOrAreaReport ID_ROOM_AREA_REPORT
SheetIssuesOrRevisions ID_SETTINGS_REVISIONS
RevisionSchedule ID_VIEW_NEW_REVISION_SCHEDULE
CalloutTags ID_VIEWTAGSTYLES_CALLOUTTAGS
ElevationTags ID_VIEWTAGSTYLES_ELEVATIONTAGS
SectionTags ID_VIEWTAGSTYLES_SECTIONTAGS
FloorByFaceFloor ID_FLOOR_PICK_FACES
HideCategory ID_VIEW_HIDE_CATEGORY
RunInterferenceCheck ID_INTERFERENCE_CHECK
SpecifyCoordinatesAtPoint ID_SPECIFY_SHARED_COORDINATES
ExportIFC ID_EXPORT_IFC
SystemBrowser ID_RBS_SYSTEM_NAVIGATOR
ResetAnalyticalModel ID_RESET_ANALYTICAL_MODEL
ExportOptionsIFCOptions ID_IFC_OPTIONS
ExportDWFOrDWFx ID_EXPORT_DWF
ExportCADFormatsDWG ID_EXPORT_DWG
ExportCADFormatsDXF ID_EXPORT_DXF
ExportCADFormatsDGN ID_EXPORT_DGN
ExportCADFormatsACIS_SAT ID_EXPORT_SAT
ExportPDF ID_EXPORT_PDF
ExportCADFormatsSTL ID_EXPORT_STL
ExportCADFormatsOBJ ID_EXPORT_OBJ
OpenIFC ID_IMPORT_IFC
RelinquishAllMine ID_RELINQUISH_ALL_MINE
MaterialTakeoff ID_VIEW_NEW_MATERIAL_TAKEOFF
DWFMarkup ID_MARKUPS_LOAD
ImportTypes IDC_COMBOBOX_CONTROL
ExportExportTypes ID_EXPORT_FAM_TYPES
OpenIFCOptions ID_IFC_IMPORT_OPTIONS
CheckMemberSupports ID_COMMAND_CHECK_MEMBER_SUPPORTS
AnalyticalConsistencyChecks ID_COMMAND_ANALYTICAL_CONSISTENCY_CHECKS
KeynoteLegend ID_VIEW_NEW_KEYNOTE_LEGEND
KeynotingSettings ID_SETTINGS_KEYNOTING
OpenBuildingComponent ID_OPEN_ADSK
Web ID_TRUSS_WEB_CURVE
TopChord ID_TRUSS_TOP_CHORD_CURVE
BottomChord ID_TRUSS_BOTTOM_CHORD_CURVE
ShapeStatus ID_SHAPE_STATUS
TogglePropertiesPalette ID_TOGGLE_PROPERTIES_PALETTE
CoordinationModel ID_COORDINATION_MODEL
StatusBarWorksets ID_STATUSBAR_WORKSETS
StatusBarDesignOptions ID_STATUSBAR_DESIGNOPTIONS
ReinforcementNumbers ID_NUMBERING_REINF_PARTITIONS
FamilyCategoryAndParameters ID_OBJECTS_FAMILYHOST
ExportImagesandAnimationsWalkthrough ID_RM_ANIMATION
ExportImagesandAnimationsSolarStudy ID_FILE_EXPORT_SOLAR_STUDY
SunSettings ID_SETTINGS_SUNANDSHADOWSSETTINGS
Grid ID_OBJECTS_GRID
ArchitecturalWall ID_OBJECTS_WALL
Door ID_OBJECTS_DOOR
Window ID_OBJECTS_WINDOW
Delete ID_BUTTON_DELETE
Space ID_OBJECTS_MEP_SPACE
SpaceTag ID_OBJECTS_MEP_SPACE_TAG
SpaceSeparator ID_OBJECTS_MEP_SPACE_SEPARATION
TypeProperties ID_EDIT_TYPE
Level ID_OBJECTS_LEVEL
FramingElevation ID_BUTTON_BRACING_ELEVATION
ArchitecturalFloor ID_OBJECTS_FLOOR
Stair ID_OBJECTS_STAIRS
ArchitecturalColumn ID_OBJECTS_COLUMN
MirrorProject ID_MIRROR_PROJECT
RotateProjectNorth ID_ROTATE_PROJECT
Rotate ID_EDIT_ROTATE
MirrorPickAxis ID_EDIT_MIRROR
LineWeights ID_SETTINGS_PENS
ObjectStyles ID_SETTINGS_OBJECTSTYLES
AnalysisDisplayStyles ID_SETTINGS_ANALYSIS_DISPLAY_STYLES
Snaps ID_SETTINGS_SNAPPING
ProjectUnits ID_SETTINGS_UNITS
StructuralPlan ID_VIEW_NEW_STRUCTURAL_PLAN
FloorPlan ID_VIEW_NEW_PLAN
Section ID_VIEW_NEW_SECTION
Camera ID_VIEW_NEW_3DVIEW
ProjectBrowser ID_VIEW_PROJECTEXPLORER
NewSheet ID_VIEW_NEW_SHEET
ImportCAD ID_FILE_IMPORT
LinkCAD ID_FILE_CADFORMAT_LINK
PointCloud ID_POINT_CLOUD
NewFamily ID_FAMILY_NEW
LoadShapes ID_FAMILY_LOAD
NewConceptualMass ID_NEW_REVIT_DESIGN_MODEL
LoadAutodeskFamily ID_FAMILY_LOAD_CLOUD
Pin ID_LOCK_ELEMENTS
Walkthrough ID_VIEW_NEW_WALKTHROUGH
Unpin ID_UNLOCK_ELEMENTS
SolidExtrusion ID_OBJECTS_EXTRUSION
PlaceAComponent ID_OBJECTS_FAMSYM
ModelLine ID_OBJECTS_PROJECT_CURVE
StairPath ID_OBJECTS_STAIRS_PATH
ReferencePlane ID_OBJECTS_CLINE
Redo ID_BUTTON_REDO
Undo ID_BUTTON_UNDO
Control ID_OBJECTS_CONTROL
PickAPlane ID_PICK_WORKPLANE
Filters ID_SETTINGS_FILTERS
TemporaryDimensions ID_SETTINGS_DIMENSIONS
LinePatterns ID_SETTINGS_PEN_FONTS
Array ID_EDIT_CREATE_PATTERN
Move ID_EDIT_MOVE
Copy ID_EDIT_MOVE_COPY
PlaceView ID_VIEW_PLACE_VIEW
Text ID_OBJECTS_TEXT_NOTE
NewTitleBlock ID_TITLEBLOCK_NEW
AutomaticCeiling ID_OBJECTS_CEILING
FillPatterns ID_SETTINGS_FILLPATTERNS
Default3DView ID_VIEW_DEFAULT_3DVIEW
Label ID_OBJECTS_TAG_NOTE
VisibilityOrGraphics ID_VIEW_CATEGORY_VISIBILITY
Opening ID_OBJECTS_OPENING
NewProject ID_FILE_NEW_CHOOSE_TEMPLATE
ActivateView ID_VIEWPORT_ACTIVATE
Materials ID_SETTINGS_MATERIALS
DeactivateView ID_VIEWPORT_ACTIVATE_BASE
MaterialAssets ID_SETTINGS_RENDERING_APPEARANCE
DecalTypes ID_SETTINGS_DECAL_TYPES
Options ID_OPTIONS
Room ID_OBJECTS_ROOM
CurtainGrid ID_OBJECTS_CW_GRID
CurtainWallMullion ID_OBJECTS_MULLION
FamilyTypes ID_FAMILY_TYPE
FilledRegion ID_OBJECTS_FILLED_REGION
RoomTag ID_OBJECTS_ROOM_TAG
Align ID_ALIGN
Symbol ID_OBJECTS_FAM_ANN_INST
DuplicateView ID_PRJBROWSER_COPY
ExportOptionsExportSetupsDGN ID_SETTINGS_EXPORT_SETUPS_DGN
ExportOptionsExportSetupsDWGOrDXF ID_SETTINGS_EXPORT_SETUPS_DWGDXF
ColorFillLegend ID_OBJECTS_ROOM_FILL
BuildingElevation ID_BUTTON_INTERIOR_ROOM_ELEVATION
JoinOrUnjoinRoof ID_JOIN_ROOF
SolidBlend ID_OBJECTS_BLEND
ManageLinks ID_LINKED_DWG
SolidRevolve ID_OBJECTS_REVOLUTION
ScheduleOrQuantities ID_VIEW_NEW_SCHEDULE
Callout ID_VIEW_NEW_CALLOUT
SolidSweep ID_OBJECTS_SWEEP
CreateGroup ID_EDIT_GROUP
Ramp ID_OBJECTS_RAMP
RoomSeparator ID_OBJECTS_AREA_SEPARATION
AlignedToCurrentView ID_EDIT_PASTE_ALIGNED
AlignedToPickedLevel ID_EDIT_PASTE_ALIGNED_PICK_LEVEL
WallJoins ID_EDIT_WALL_JOINS
StartingView ID_STARTING_VIEW
AlignedToSelectedLevels ID_EDIT_PASTE_ALIGNED_LEVEL_BY_NAME
TagByCategory ID_BUTTON_TAG
AlignedToSelectedViews ID_EDIT_PASTE_ALIGNED_VIEWS_BY_NAME
LoadedTagsAndSymbols ID_SETTINGS_TAGS
NewAnnotationSymbol ID_ANNOTATION_SYMBOL_NEW
AlignedToSamePlace ID_EDIT_PASTE_ALIGNED_SAME_PLACE
SpotSlopeTypes ID_SETTINGS_SPOT_SLOPE
Arrowheads ID_SETTINGS_ANNOTATIONS_LEADERS
SpotElevationTypes ID_SETTINGS_SPOT_ELEVATION
SpotCoordinateTypes ID_SETTINGS_SPOT_COORDINATE
ReviewWarnings ID_REVIEW_WARNINGS
AlignmentStationLabelTypes ID_SETTINGS_ALIGNMENT_STATION_LABEL
LineStyles ID_SETTINGS_LINE_STYLES
ReflectedCeilingPlan ID_VIEW_NEW_RCP
DetailLevel ID_DETAIL_LEVEL
VoidExtrusion ID_OBJECTS_EXTRUSION_CUT
VoidBlend ID_OBJECTS_BLEND_CUT
VoidRevolve ID_OBJECTS_REVOLUTION_CUT
VoidSweep ID_OBJECTS_SWEEP_CUT
CutGeometry ID_CUT_HOST
UncutGeometry ID_UNCUT_HOST
PlaceDetailGroup ID_OBJECTS_PLACE_DETAIL_GROUP
OverrideByCategory ID_VIEW_OVERRIDE_CATEGORY
DetailComponent ID_OBJECTS_FAMDETAIL
DetailLine ID_OBJECTS_DETAIL_CURVES
Insulation ID_OBJECTS_INSULATION
CreateSimilar ID_EDIT_CREATE_SIMILAR
Worksets ID_SETTINGS_PARTITIONS
Phases ID_SETTINGS_PHASES
PropertyLine ID_SITE_PROPERTYLINE
BuildingPad ID_SITE_BUILDINGPAD
ImportImage ID_OBJECTS_RASTER
ImportPDF ID_OBJECTS_RASTER_PDF
MatchTypeProperties ID_EDIT_MATCH_TYPE
ScopeBox ID_VOLUME_OF_INTEREST
Linework ID_EDIT_LINEWORK
SetWorkPlane ID_SKETCH_PLANE_TOOL
DraftingView ID_VIEW_NEW_DRAFTING
Legend ID_VIEW_NEW_LEGEND
Demolish ID_EDIT_DEMOLISH
RevisionCloud ID_OBJECTS_CLOUD
SynchronizeAndModifySettings ID_FILE_SAVE_TO_CENTRAL
ProjectInformation ID_SETTINGS_PROJECT_INFORMATION
ModelInPlace ID_INPLACE_COMPONENT
SheetList ID_VIEW_NEW_DRAWING_LIST
AreaPlan ID_VIEW_NEW_AREASCHEME
GradedRegion ID_SITE_GRADE
Toposurface ID_SITE_TOPO_SURFACE
ManageImages ID_EDIT_RASTER_SYMBOLS
ApplyTemplatePropertiesToCurrentView ID_APPLY_VIEW_TEMPLATE
RevealWall ID_OBJECTS_REVEAL
SweepWall ID_OBJECTS_CORNICE
Paint ID_EDIT_PAINT
RemovePaint ID_EDIT_UNPAINT
NoteBlock ID_VIEW_NEW_NOTE_BLOCK
ModelText ID_OBJECTS_MODELTEXT
ShowHiddenLinesByElement ID_HIDE_ELEMENTS_EDITOR
RemoveHiddenLinesByElement ID_UNHIDE_ELEMENTS_EDITOR
ManageViewTemplates ID_SETTINGS_VIEWTEMPLATES
CreateTemplateFromCurrentView ID_CREATE_VIEW_TEMPLATE
SplitFace ID_SPLIT_FACE
RestoreBackup ID_FILE_BACKUPS
ExportODBCDatabase ID_FILE_EXPORT_ODBC
LoadAsGroup ID_LOAD_GROUP
SaveAsLibraryGroup ID_SAVE_GROUP
ParkingComponent ID_SITE_PARKING_COMPONENT
TransferProjectStandards ID_TRANSFER_PROJECT_STANDARDS
SymbolicLine ID_OBJECTS_VIEW_DIR_SPEC_CURVE
CutProfile ID_EDIT_CUT_BOUNDARY
ExportReportsSchedule ID_FILE_EXPORT_SCHEDULE
CloseInactiveViews ID_VIEW_CLOSE_INACTIVE
AreaTag ID_OBJECTS_AREA_TAG
AreaBoundary ID_OBJECTS_AREASCHEME_BOUNDARY
Area ID_OBJECTS_AREA
JoinGeometry ID_JOIN_ELEMENTS_EDITOR
UnjoinGeometry ID_UNJOIN_ELEMENTS_EDITOR
SwitchJoinOrder ID_SWITCH_JOIN_ORDER_EDITOR
TagAllNotTagged ID_BUTTON_TAG_ALL
MergeSurfaces ID_MERGE_SURFACE
SharedParameters ID_FILE_EXTERNAL_PARAMETERS
LegendComponent ID_OBJECTS_LEGEND_COMPONENT
Offset ID_OFFSET
PurgeUnused ID_PURGE_UNUSED
PlaceDecal ID_RM_CREATE_DECAL
TitleBlock ID_OBJECTS_PLACE_TITLEBLOCK
RebarLine ID_OBJECTS_REBAR_CURVE
LabelContours ID_SITE_LABEL_CONTOURS
SplitWithGap ID_SPLIT_WITH_GAP
ThinLines ID_THIN_LINES
LinkRevit ID_RVTDOC_LINK
ExportImagesandAnimationsImage ID_FILE_PRINT_TO_IMAGE
ProjectParameters ID_SETTINGS_PROJECT_PARAMETERS
RelocateProject ID_RELOCATE_SHARED_COORDINATES
RotateTrueNorth ID_ROTATE_SHARED_COORDINATES
DuplicateWithDetailing ID_DUPLICATE_WITH_DETAILING
Location ID_GEO_MANAGE_LOCATIONS
AcquireCoordinates ID_GEO_ACQUIRE_COORDINATES
ReportSharedCoordinates ID_REPORT_SHARED_COORDINATES
IdsOfSelection ID_IDS_OF_SELECTION
SelectById ID_SELECT_BY_ID
PublishCoordinates ID_GEO_PUBLISH_COORDINATES
ParametersService ID_SETTINGS_FORGE_PARAMETERS
PlanRegion ID_NEW_PLAN_REGION
BrowserOrganization ID_BROWSER_ORGANIZATION
Matchline ID_NEW_MATCHLINE
ViewReference ID_NEW_REFERENCE_VIEWER
DesignOptions ID_EDIT_DESIGNOPTIONS
AddToSet ID_EDIT_ADDTODESIGNOPTIONSET
PickToEdit ID_EDIT_PICKS_OPTION
EditingRequests ID_WORKSET_EDITING_REQUESTS
SaveAsLibraryFamily ID_SAVE_FAMILY_ANY
Subregion ID_SITE_AREA
Railing ID_OBJECTS_RAILING
Beam ID_OBJECTS_BEAM
Brace ID_OBJECTS_BRACE
StructuralColumn ID_OBJECTS_STRUCTURAL_COLUMN
StructuralWall ID_OBJECTS_STRUCTURAL_WALL
SpanDirectionSymbol ID_OBJECTS_SPAN_DIR_SYM
StructuralFloor ID_OBJECTS_SLAB
Scale ID_EDIT_SCALE
Loads ID_OBJECT_LOADS
StructuralSettings ID_STRUCTURAL_SETTINGS
ReferenceLine ID_OBJECTS_REFERENCE_CURVE
RebarCoverSettings ID_REBAR_COVER_SETTINGS
ShowMassFormAndFloors ID_SHOW_MASS_STANDARD
CurtainSystemByFace ID_CURTA_SYSTEM_PICK_FACES
WallByFaceWall ID_WALL_PICK_FACES
ShowMassByViewSettings ID_SHOW_MASS_NO_OVERRIDE
ShowMassSurfaceTypes ID_SHOW_MASS_SURFACES
ShowMassZonesAndShades ID_SHOW_MASS_ZONES
StructuralRebar ID_REBAR_PLACE
CopyMonitorUseCurrentProject ID_COPY_WATCH_CURRENT
CopyMonitorSelectLink ID_COPY_WATCH_LINK
CoordinationReviewUseCurrentProject ID_RECONCILE_CURRENT
CoordinationSelectLink ID_RECONCILE_LINK
Wall ID_OBJECTS_CONTINUOUS_FOOTING
Isolated ID_OBJECTS_ISOLATED_FOOTING
MaterialTag ID_TAG_MATERIAL
CoordinationSettings ID_COPY_SETTINGS
Slab ID_OBJECTS_FOOTING_SLAB
ElementKeynote ID_KEYNOTE_ELEMENT
MaterialKeynote ID_KEYNOTE_MATERIAL
UserKeynote ID_KEYNOTE_USER
LoadSelection ID_RETRIEVE_FILTERS
SaveSelection ID_FILTERS_SELECTION_SAVE
EditSelection ID_EDIT_SELECTIONS
ActivateControlsAndDimensions ID_TOGGLE_ACTIVATE_CONTROLS
ShowWorksharingMakeEditableControls ID_TOGGLE_SHOW_MAKE_ELEMENT_EDITABLE_CONTROLS
MultiCategoryTag ID_TAG_MULTI
BoundaryConditions ID_OBJECT_BC
StructuralPathReinforcement ID_PATH_REIN_SKETCH
PathReinforcementSymbol ID_OBJECTS_PATH_REIN_SPAN_SYM
OpeningByFace ID_CREATE_OPENING_BY_FACE_TB
VerticalOpening ID_CREATE_VERTICAL_OPENING_TB
DormerOpening ID_CREATE_DORMER_OPENING_TB
WallOpening ID_CREATE_WALL_OPENING_TB
ShaftOpening ID_CREATE_SHAFT_OPENING_TB
SpotSlope ID_SPOT_SLOPE
HideElements ID_VIEW_HIDE_ELEMENTS
ToggleRevealHiddenElementsMode ID_VIEW_FRAME_REVEALHIDDEN
OverrideByElement ID_VIEW_OVERRIDE_ELEMENTS
OverrideByFilter ID_VIEW_GO_FILTER
DuplicateAsDependent ID_CREATE_DEPENDENT_VIEW
MaskingRegion ID_MASKING_REGION
MajorSegment ID_MAJOR_SEGMENT
ReinforcementSettings ID_REBAR_ABBREVIATION
ExportFBX ID_EXPORT_FBX
OpenFamily ID_FAMILY_OPEN
CreateParts ID_CREATE_PARTS
ViewCube ID_SHOW_VIEWCUBE
BeamAnnotations ID_BEAM_ANNOTATION_PLACEMENT
AllowableBarTypes ID_REBAR_SHAPE_ALLOWABLE_BAR_TYPES
MacroManager ID_TOOLS_MACROS
MacroSecurity ID_TOOLS_MACROSECURITY
NavigationBar ID_NAVIGATION_BAR
FormWorkPlaneView ID_WORKPLANE_VIEW
ReconcileHosting ID_TOGGLE_HOST_BY_LINK_BROWSER
AdjustAnalyticalModel ID_AM_EDIT_MODE_START
DiameterDimension ID_ANNOTATIONS_DIMENSION_DIAMETER
DiameterDimensionTypes ID_SETTINGS_DIMENSIONS_DIAMETER
StructuralFabricArea ID_FABRIC_DISTR_SYSTEM_SKETCH
FabricReinforcementSymbol ID_FABRIC_SYSTEM_PLACE_SPANSYMBOL
PlaceOnStairOrRamp ID_OBJECTS_RAILING_ON_HOST
DuctPressureLossReport ID_MEP_DUCT_PRESSURE_LOSS_REPORT
PipePressureLossReport ID_MEP_PIPE_PRESSURE_LOSS_REPORT
OpenSampleFiles ID_SAMPLES_OPEN
SingleFabricSheetPlacement ID_FABRIC_SINGLE_PLACEMENT
AlignedMultiRebarAnnotation ID_MULTI_REFERENCE_ANNOTATION_ALIGNED
LinearMultiRebarAnnotation ID_MULTI_REFERENCE_ANNOTATION_LINEAR
FabricationSettings ID_MEP_FABRICATION_SETTINGS
LinkIFC ID_IFC_LINK
SelectionBox ID_VIEW_APPLY_SELECTION_BOX
StructuralRebarCoupler ID_REBAR_COUPLER
PAndIDModeler ID_MEP_PNID_MODELER
PAndIDSettings ID_MEP_PNID_SETTINGS
TogglePAndIDModelerBrowser ID_MEP_TOGGLE_PNID_BROWSER
DuctAccessory ID_RBS_MECHANICAL_ACCESSORY
AirTerminal ID_RBS_MECHANICAL_DIFFUSER
Duct ID_RBS_MECHANICAL_DUCT
MechanicalEquipment ID_RBS_MECHANICAL_EQUIPMENT
DuctFitting ID_RBS_MECHANICAL_FITTING
FlexDuct ID_RBS_MECHANICAL_FLEX
ConvertToFlexDuct ID_RBS_MECHANICAL_CONVERTTOFLEX
DuctLegend ID_RBS_MECHANICAL_COLOR_FILL
PanelSchedules ID_RBS_VIEW_PANEL_SCHEDULE
ElectricalSettings ID_RBS_ELECTRICAL_SETTINGS
LoadClassifications ID_RBS_ELECTRICAL_LOAD_CLASSIFICATION
LightingFixture ID_RBS_LIGHTING_FIXTURE
ElectricalEquipment ID_RBS_ELECTRICAL_EQUIPMENT
ElectricalFixture ID_RBS_ELECTRICAL_DEVICE
CheckCircuits ID_RBS_CHECK_CIRCUITS
DemandFactors ID_RBS_ELECTRICAL_DEMAND_FACTOR
Pipe ID_RBS_PIPE_PIPE
FlexPipe ID_RBS_PIPE_FLEX
PipeFitting ID_RBS_PIPE_FITTING
PipeAccessory ID_RBS_PIPE_ACCESSORY
ArcWire ID_RBS_ELECTRICAL_WIRE
PipeLegend ID_RBS_PIPING_COLOR_FILL
CheckPipeSystems ID_RBS_CHECK_PIPE_SYSTEMS
CheckDuctSystems ID_RBS_CHECK_DUCT_SYSTEMS
MechanicalSettings ID_RBS_MECHANICAL_SETTINGS
PlumbingFixture ID_RBS_PLUMBING_FIXTURE
Sprinkler ID_RBS_SPRINKLER
ElectricalConnector ID_RBS_ADD_ELECTRICAL_CONNECTOR
DuctConnector ID_RBS_ADD_DUCT_CONNECTOR
PipeConnector ID_RBS_ADD_PIPE_CONNECTOR
Zone ID_RBS_HVAC_ZONE
BuildingOrSpaceTypeSettings ID_RBS_BUILDINGSPACE_TYPE_SETTINGS
ChamferedWire ID_RBS_ELECTRICAL_WIRE_CHAMFER
SplineWire ID_RBS_ELECTRICAL_WIRE_SPLINE
Communication ID_RBS_ELECTRICAL_COMMUNICATION_DEVICE
Data ID_RBS_ELECTRICAL_DATA_DEVICE
FireAlarm ID_RBS_ELECTRICAL_FIREALARM_DEVICE
Lighting ID_RBS_ELECTRICAL_LIGHTING_DEVICE
NurseCall ID_RBS_ELECTRICAL_NURSECALL_DEVICE
Security ID_RBS_ELECTRICAL_SECURITY_DEVICE
Telephone ID_RBS_ELECTRICAL_TELEPHONE_DEVICE
CableTrayConnector ID_RBS_ADD_CABLETRAY_CONNECTOR
ConduitConnector ID_RBS_ADD_CONDUIT_CONNECTOR
CableTrayFitting ID_RBS_CABLETRAY_FITTING
ConduitFitting ID_RBS_CONDUIT_FITTING
CableTray ID_RBS_CABLE_TRAY
Conduit ID_RBS_CONDUIT
ManageTemplates ID_RBS_PANEL_SCHEDULE_MANAGE_TEMPLATES
EditATemplate ID_RBS_PANEL_SCHEDULE_EDIT_A_TEMPLATE
ParallelConduits ID_PARALLEL_CONDUIT
DuctPlaceholder ID_DUCT_1LINE_PATH
PipePlaceholder ID_PIPE_1LINE_PATH
ParallelPipes ID_PARALLEL_PIPES
ShowDisconnects ID_SHOWDISCONNECTS_BUTTON
MultiPointRouting ID_FABRICATION_PART_START_MPR
SystemZone ID_MEP_CREATE_SYSTEM_ZONE
SiteComponent ID_SITE_COMPONENT
AreaAndVolumeComputations ID_SETTING_AREACALCULATIONS
ShowWorkPlane ID_SKETCH_GRID_VIS
Fascia ID_CREATE_FASCIA_TB
Gutter ID_CREATE_GUTTER_TB
SlabEdgeFloor ID_CREATE_SLAB_EDGE_TB
CheckSpelling ID_CHECK_SPELLING
RepeatingDetailComponent ID_OBJECTS_REPEATING_DETAIL
InsertViewsFromFile ID_INSERT_VIEWS_FROM_FILE
Insert2DElementsFromFile ID_INSERT_2D_ELEMENTS_FROM_FILE
SaveAsLibraryView ID_SAVE_VIEWS_TO_FILE
AutomaticBeamSystem ID_OBJECTS_JOIST_SYSTEM
PlaceMass ID_OBJECTS_MASS
InPlaceMass ID_INPLACE_MASS
SynchronizeNow ID_FILE_SAVE_TO_CENTRAL_SHORTCUT
StructuralTrusses ID_OBJECTS_TRUSS
CreateAnAreaBasedLoad IDS_RBS_ANALYTICAL_LOADZONE
AreaBasedLoadBoundary ID_RBS_ANALYTICAL_AREA_BASED_LOAD_BOUNDARY
EquipmentLoad ID_RBS_ELECTRICAL_ANALYSIS_ADD_EQUIPMENT_LOAD
ShowBoundaryOpenEnds ID_RBS_SHOW_ABL_SEPARATION_LINE_OPEN_END
MechanicalControlDevice ID_RBS_MECHANICAL_CONTROL_DEVICE
PlumbingEquipment ID_RBS_PLUMBING_EQUIPMENT
HideBoundaryOpenEnds ID_RBS_HIDE_ABL_SEPARATION_LINE_OPEN_END
ShowLastReport ID_INTERFERENCE_REPORT
GraphicalColumnSchedule ID_VIEW_NEW_GRAPH_SCHED_COLUMN
ApplyCoping ID_COPING
RemoveCoping ID_UNCOPING
BeamSystemSymbol ID_OBJECTS_BEAM_SYSTEM_TAG
StructuralAreaReinforcement ID_REBAR_SYSTEM_SKETCH
AreaReinforcementSymbol ID_OBJECTS_REBAR_SYSTEM_SPAN_SYM
SolidSweptBlend ID_OBJECTS_SWEPTBLEND
VoidSweptBlend ID_OBJECTS_SWEPTBLEND_CUT
EditRebarCover ID_CONCRETE_COVER
ColorSchemes ID_SETTINGS_COLORFILLSCHEMES
CreateAnalyticalLink ID_CREATE_ANALYTICAL_LINK
TrimOrExtendToCorner ID_TRIM_EXTEND_CORNER
TrimOrExtendSingleElement ID_TRIM_EXTEND_SINGLE
TrimOrExtendMultipleElements ID_TRIM_EXTEND_MULTIPLE
AlignedDimension ID_ANNOTATIONS_DIMENSION_ALIGNED
LinearDimension ID_ANNOTATIONS_DIMENSION_LINEAR
AngularDimension ID_ANNOTATIONS_DIMENSION_ANGULAR
RadialDimension ID_ANNOTATIONS_DIMENSION_RADIAL
ArcLengthDimension ID_ANNOTATIONS_DIMENSION_ARCLENGTH
MeasureBetweenTwoReferences ID_MEASURE_LINE
MeasureAlongAnElement ID_MEASURE_PICK_LINES
MirrorDrawAxis ID_EDIT_MIRROR_LINE
KeyboardShortcuts ID_KEYBOARD_SHORTCUT_DIALOG
BeamOrColumnJoins ID_EDIT_BEAM_JOINS
SaveAsTemplate ID_REVIT_SAVE_AS_TEMPLATE
SaveAsFamily ID_REVIT_SAVE_AS_FAMILY
HalftoneOrUnderlay ID_SETTINGS_HALFTONE
Multiplanar ID_TURN_ON_MULTI_PLANAR_SHAPE
OpenProject ID_APPMENU_PROJECT_OPEN
LoadCases ID_STRUCTURAL_SETTINGS_LOAD_CASES
LoadCombinations ID_STRUCTURAL_SETTINGS_LOAD_COMB
BoundaryConditionsSettings ID_STRUCTURAL_SETTINGS_BOUND_COND
FindOrReplace ID_FIND_REPLACE
EnergySettings ID_CEA_VIEW_ENERGY_DATA
Generate ID_CEA_LAUNCH_ANALYSIS
ExportGBXML ID_EXPORT_GBXML
GuideGrid ID_CREATE_GUIDE_GRID
CreateAssembly ID_CREATE_ASSEMBLY
StairTreadOrRiserNumber ID_STAIRS_TRISER_NUMBER
ManageConnectionToARevitServerAccelerator ID_MANAGE_CONNECTION_TO_A_REVIT_SERVER_ACCELERATOR
RenderInCloud ID_PHOTO_RENDER_IN_CLOUD
RenderGallery ID_PHOTO_RENDER_GALLERY
CreateEnergyModel ID_ENABLE_ENERGY_ANALYSIS
DisplaceElements ID_DISPLACEMENT_CREATE
GlobalParameters ID_SETTINGS_GLOBAL_PARAMETERS
Collaborate ID_COLLABORATE
CollaborateInCloud ID_COLLABORATE_360
PublishSettings ID_VIEWS_FOR_A360
AssemblyCode ID_SETTINGS_ASSEMBLY_CODE
RevealObstacles ID_PATH_OF_TRAVEL_SHOW_OBSTACLES
PathOfTravel ID_PATH_OF_TRAVEL
MultipleValuesIndication ID_MULTIPLE_VALUES_INDICATOR_SETTINGS
MultiplePaths ID_MULTIPLE_PATHS
OneWayIndicator ID_ONE_WAY_INDICATOR
PeopleContent ID_PEOPLE_CONTENT
SpatialGrid ID_SPATIAL_GRID
LoadFamilyIntoProjectAndClose ID_LOAD_INTO_PROJECTS_CLOSE
FabricationPart ID_TOGGLE_FABPART_BROWSER
LoadRebarShapeIntoProjectAndClose ID_LOAD_INTO_PROJECTS_CLOSE_REBAR_SHAPE
Dynamo ID_VISUAL_PROGRAMMING_DYNAMO
DeleteEnergyModel ID_DISABLE_ENERGY_ANALYSIS
Shorten ID_CREATE_EXT_ENTITY_SHORTENING
CopeSkewed ID_CREATE_EXT_ENTITY_BEAM_COPE
CornerCut ID_CREATE_EXT_ENTITY_CORNER_CUT
Welds ID_CREATE_EXT_ENTITY_WELD
Plate ID_CREATE_EXT_ENTITY_PLATE
Bolts ID_CREATE_EXT_ENTITY_BOLT
Holes ID_CREATE_EXT_ENTITY_HOLE
Anchors ID_CREATE_EXT_ENTITY_ANCHOR
ShearStuds ID_CREATE_EXT_ENTITY_SHEAR_STUD
ContourCut ID_CREATE_EXT_ENTITY_CONTOUR
Cope ID_STEEL_PARAM_CUT_COPE
Miter ID_STEEL_PARAM_CUT_MITER
SawCutFlange ID_STEEL_PARAM_CUT_SAW_CUT_FLANGE
SawCutWeb ID_STEEL_PARAM_CUT_SAW_CUT_WEB
CutThrough ID_STEEL_PARAM_CUT_THROUGH
CutBy ID_STEEL_PARAM_CUT_BY
ToggleHome ID_REVIT_MODEL_BROWSER
SaveAsCloudModel ID_REVIT_FILE_SAVE_AS_CLOUD_MODEL
SystemsAnalysis ID_RUN_SYSTEMS_ANALYSIS
LinkPDF ID_LINK_PDF
LinkImage ID_LINK_IMAGE
AnalyticalMember ID_ANALYTICAL_MODEL_MEMBERS
BatchPrint ID_BATCH_PRINT
WorksharingMonitor ID_WORKSHARING_MONITOR
SharedViews ID_SHARED_VIEWS
ResetSharedCoordinates ID_RESET_SHARED_COORDINATES
ElectricalAnalyticalLoadTypeSettings ID_MEP_ELECTRICAL_ANALYSIS_SETTINGS
GenerateAnalysis ID_SYSTEMS_ANALYSIS_INSIGHT
ViewAnalysis ID_SYSTEMS_ANALYSIS_RESULTS_INSIGHT
OpenCloudModel ID_FILE_OPEN_CLOUD_MODEL
DynamoPlayerForSteel ID_STEEL_PLAYLIST_DYNAMO
PanelByBoundary ID_CREATE_ANALYTICAL_MODEL_PANEL
AnalyticalAutomation ID_ANALYTICAL_AUTOMATION_PLAYLIST_DYNAMO
PanelByExtrusion ID_CREATE_ANALYTICAL_MODEL_PANEL_BY_EXTRUSION
SpaceNaming ID_MEP_SPACE_NAMING_UTILITY
DynamoPlayer ID_PLAYLIST_DYNAMO
RepairCentralModel ID_REPAIR_CENTRAL_MODEL
LinkTopography ID_TOPOGRAPHY_LINK
Optimize ID_CEA_OPTIMIZE
OpenRevitFile ID_REVIT_FILE_OPEN
Close ID_REVIT_FILE_CLOSE
Save ID_REVIT_FILE_SAVE
SaveAsProject ID_REVIT_FILE_SAVE_AS
PrintSetup ID_REVIT_FILE_PRINT_SETUP
Print ID_REVIT_FILE_PRINT
PrintPreview ID_REVIT_FILE_PRINT_PREVIEW
CopyToClipboard ID_EDIT_COPY
CutToClipboard ID_EDIT_CUT
PasteFromClipboard ID_EDIT_PASTE
TabViews ID_VIEW_TAB
TileViews ID_WINDOW_TILE_VERT
ExitRevit ID_APP_EXIT
StatusBar ID_VIEW_STATUS_BAR
You can also get this list of all these commands by using
pyRevit -> Spy -> List Elements -> System Postable Commands


How to Subscribe to Events Manually?
Lastly, here is an example on how to subscribe to events manually. pyRevit takes this step from us, and makes it even nicer to manage it in a folder. But you might want to know how it's used too.
You need to do the following steps:
1️⃣ Create a functions that will be subscribed to an event. I called it import_replacement in my example
2️⃣ Get Command Id with LookupCommandId or LookupPostableCommandId
3️⃣ CreateAddInCommandBinding
4️⃣ Now you can subscribe your function to this command. Make sure you select from BeforeExecuted, CanExecute, Executed Events.
Here is an Example:
from Autodesk.Revit import UI
from Autodesk.Revit.DB import *
import clr
clr.AddReference('System')
from System import EventHandler
def import_replacement(sender, args):
TaskDialog.Show('Stop!', 'Do not import!')
uiapp = __revit__
commandId = UI.RevitCommandId.LookupCommandId("ID_FILE_IMPORT")
try:
import_binding = uiapp.CreateAddInCommandBinding(commandId)
import_binding.BeforeExecuted += EventHandler[UI.Events.ExecutedEventArgs](import_replacement)
except:
import traceback
print(traceback.format_exc())
You can use RevitPythonShell to test it. But keep in mind that this event handler will work until you restart Revit or unsubscribe from an event. To unsubscribe from the event, you can use the same code and only change +=
to -=
like this:
import_binding.Executed -= EventHandler[UI.Events.ExecutedEventArgs](eventhandler_file_import)
Happy Coding!
Congratulations!
You've just unlocked a new achievement and you can use pyRevit Hooks and Revit API Events!