Dec 1, 2020
Get CropBox Id in Revit with Python.
Learn how to get CropBox Id in Revit API.
1️⃣ FilteredElementCollector.Excluding()
The first method is quite straight forward and goes like this:
Hide the CropBox
Use FilteredElementCollector(doc, view_id) to collect all elements you can see in the view.
Unhide the CropBox
Use FilteredElementCollector again, but this time exclude elements you collected the first time with .Excluding(element_ids)
Get ElementId of the CropBox from the collection.
Lastly, RollBack your Transactions so nothing has changed.
This method is a bit messy because of multiple transactions. So I have another option.
FilteredElementCollector.WherePasses()
I have found an article by Jeremy Tammik - "Efficiently Retrieve Crop Box for Given View" in C#.
So I've translated it to python for people who don't "speak" C# well enough. I encourage you to read his article to better understand the logic behind this function.
Be aware that FilteredElementCollector will grab CropBox and View so be aware of that.
Join Newsletter
📩 You will be added to Revit API Newsletter
Join Us!
which is already read by 7500+ people!





Ready to become Revit Hero for your office? Learn Revit API!
Join this comprehensive course that will guide you step by step on how to create your dream tools for Revit that save time.
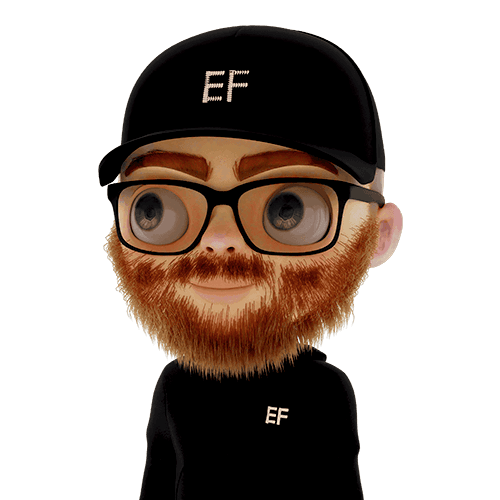