Sep 25, 2022
Revit API: How to change associated Level in Walls?
Check out this snippet with explanation to change top and base constraints of a Wall.
Get Parameters
First of all we need to have an element that we are going to modify. I am going to get a wall with an ElementId
Then we need to look inside and find parameters that we want to modify. You can do it easily with RevitLookup plugin that let's us Snoop Current Selection

Then if you click on Definition you can see BuiltInName
of this Parameter. Which is - WALL_BASE_CONSTRAINT
Then do the same to find names of Top Constraint
parameter and Unconnected Height

Since these are BuiltInParamteres
we can get them with .get_Parameter()
method
Set New Levels
To set new levels we just going to take parameter variables we have written already and use .Set()
method and provide ElementId
of new levels.
Set Wall Top Constraint to None
If you want to change Top Constraint
to None
we need to provide ElementId(-1)
instead of Level.Id
This is equivalent to None in Revit API.
Final Code:
Join Newsletter
📩 You will be added to Revit API Newsletter
Join Us!
which is already read by 7500+ people!





Ready to become Revit Hero for your office? Learn Revit API!
Join this comprehensive course that will guide you step by step on how to create your dream tools for Revit that save time.
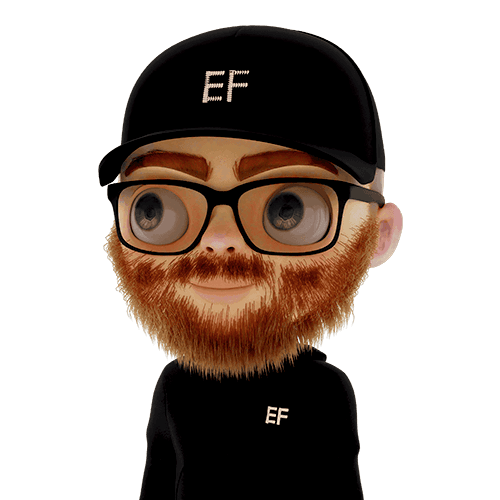